AWS SQS, Boto3 and Python: Complete Guide with examples
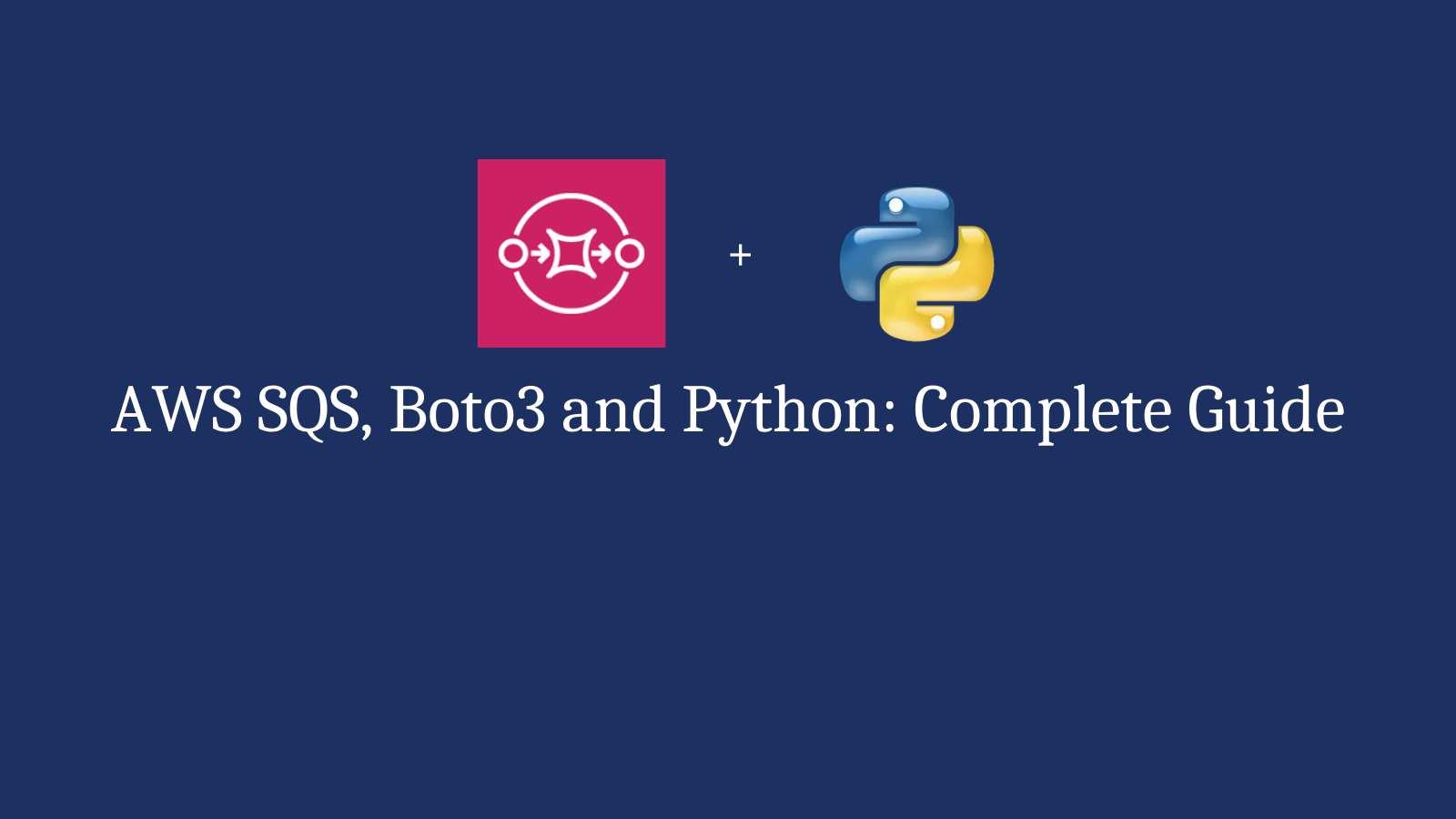
AWS Boto3 is the Python SDK for AWS. Boto3 can be used to directly interact with AWS resources from Python scripts. In this tutorial, we will look at how we can use the Boto3 library to perform various operations on AWS SQS.
Table of contents
- Prerequisites
- How to create a new SQS queue using Boto3?
- How to get the URL of SQS queue?
- How to send a message to a SQS queue?
- How to receive a message from a SQS queue?
- How to delete a message from a SQS queue?
- How to change attributes of SQS queue?
- How to remove all messages from a SQS queue?
Prerequisites
- Python3
- Boto3: Boto3 can be installed using pip:
pip install boto3
- AWS Credentials: If you haven’t setup AWS credentials before, this resource from AWS is helpful.
How to create a new SQS queue using Boto3?
We will be using the create_queue
method from Boto3 to create a new SQS queue. Some of the important parameters to keep in mind while using this method:
- QueueName: Name of the queue that you want to create
- Attributes: Specify the attribute values for the queue. Some of the commonly used attributes are:
DelaySeconds
: Messages are delayed by this value before being delivered.RedrivePolicy
: Specifies the dead-letter queue functionalityVisibilityTimeout
: Visibility timeout for the queue in seconds. This is the period of time where a particular message is only visible to a single consumer.
In the following example, we will create a queue name my-new-queue
with DelaySeconds
set to 0 and VisibilityTimeout
set to 60 seconds.
def create_queue():
sqs_client = boto3.client("sqs", region_name="us-west-2")
response = sqs_client.create_queue(
QueueName="my-new-queue",
Attributes={
"DelaySeconds": "0",
"VisibilityTimeout": "60", # 60 seconds
}
)
print(response)
Output:
{'QueueUrl': 'https://us-west-2.queue.amazonaws.com/xxxx/my-new-queue', 'ResponseMetadata': {'RequestId': '91187b97-a624-5f94-988e-23c25057248f', 'HTTPStatusCode': 200, 'HTTPHeaders': {'x-amzn-requestid': '91187b97-a624-5f94-988e-23c25057248f', 'date': 'Fri, 18 Dec 2020 00:13:39 GMT', 'content-type': 'text/xml', 'content-length': '334'}, 'RetryAttempts': 0}}
How to get the URL of SQS queue?
Most of the SQS APIs require the QueueUrl so we will use the get_queue_url
method to retrieve the URL of the queue using the QueueName
def get_queue_url():
sqs_client = boto3.client("sqs", region_name="us-west-2")
response = sqs_client.get_queue_url(
QueueName="my-new-queue",
)
return response["QueueUrl"]
Output:
https://us-west-2.queue.amazonaws.com/xxxx/my-new-queue
How to send a message to a SQS queue?
We will be using the send_message
method from Boto3 to send a message to the SQS queue. Some of the important parameters to keep in mind while using this method:
QueueUrl
: URL of the queue we want to send a message toMessageBody
: The message we want to send. The message needs to be serialized as a String.
def send_message():
sqs_client = boto3.client("sqs", region_name="us-west-2")
message = {"key": "value"}
response = sqs_client.send_message(
QueueUrl="https://us-west-2.queue.amazonaws.com/xxx/my-new-queue",
MessageBody=json.dumps(message)
)
print(response)
Output:
{'MD5OfMessageBody': '88bac95f31528d13a072c05f2a1cf371', 'MessageId': '2ce1541b-0472-4715-8375-f8a8587c16e9', 'ResponseMetadata': {'RequestId': '02a7b659-c044-5357-885e-ee0c398e24b0', 'HTTPStatusCode': 200, 'HTTPHeaders': {'x-amzn-requestid': '02a7b659-c044-5357-885e-ee0c398e24b0', 'date': 'Fri, 18 Dec 2020 00:27:54 GMT', 'content-type': 'text/xml', 'content-length': '378'}, 'RetryAttempts': 0}}
How to receive a message from a SQS queue?
We will be using the receive_message
method from Boto3 to send a message to the SQS queue. Some of the important parameters to keep in mind while using this method:
QueueUrl
: URL of the queue we want to send a message toMaxNumberOfMessages
: The maximum number of messages to retrieve.WaitTimeSeconds
: Amount of time to wait for a message to arrive in the queue. Useful for long-polling of messages.
def receive_message():
sqs_client = boto3.client("sqs", region_name="us-west-2")
response = sqs_client.receive_message(
QueueUrl="https://us-west-2.queue.amazonaws.com/xxx/my-new-queue",
MaxNumberOfMessages=1,
WaitTimeSeconds=10,
)
print(f"Number of messages received: {len(response.get('Messages', []))}")
for message in response.get("Messages", []):
message_body = message["Body"]
print(f"Message body: {json.loads(message_body)}")
print(f"Receipt Handle: {message['ReceiptHandle']}")
Output:
Number of messages received: 1
Message body: {'key': 'value'}
How to delete a message from a SQS queue?
It is important to keep in mind that receiving a message from the SQS queue doesn’t automatically delete it. Any other consumer can also retrieve the same message once the VisibilityTimeout
period expires. To ensure, no other consumer retrieves the same message, it needs to be deleted within the VisibilityTimeout
time period.
We will be using delete_message
to delete the message from the SQS queue.
- We need to provide the
ReceiptHandle
as an argument to thedelete_message
method.
def delete_message(receipt_handle):
sqs_client = boto3.client("sqs", region_name="us-west-2")
response = sqs_client.delete_message(
QueueUrl="https://us-west-2.queue.amazonaws.com/xxx/my-new-queue",
ReceiptHandle=receipt_handle,
)
print(response)
Output
{'ResponseMetadata': {'RequestId': 'd9a860cb-45ff-58ec-8232-389eb8d7c2c6', 'HTTPStatusCode': 200, 'HTTPHeaders': {'x-amzn-requestid': 'd9a860cb-45ff-58ec-8232-389eb8d7c2c6', 'date': 'Fri, 18 Dec 2020 00:42:16 GMT', 'content-type': 'text/xml', 'content-length': '215'}, 'RetryAttempts': 0}}
How to change attributes of SQS queue?
There is often a need to change SQS attributes like the VisiblityTimeout
or DelaySeconds
after the queue has already been created. We can use the set_queue_attributes
method to change the attributes.
def change_queue_attributes():
sqs_client = boto3.client("sqs", region_name="us-west-2")
response = sqs_client.set_queue_attributes(
QueueUrl="https://us-west-2.queue.amazonaws.com/734066626836/my-new-queue",
Attributes={
"DelaySeconds": "10",
"VisibilityTimeout": "300",
}
)
print(response)
---
Output:
```bash
{'ResponseMetadata': {'RequestId': '89461462-8566-5a06-b9e8-4c377b18016f', 'HTTPStatusCode': 200, 'HTTPHeaders': {'x-amzn-requestid': '89461462-8566-5a06-b9e8-4c377b18016f', 'date': 'Fri, 18 Dec 2020 00:47:59 GMT', 'content-type': 'text/xml', 'content-length': '225'}, 'RetryAttempts': 0}}
How to remove all messages from a SQS queue?
Boto3 provides the purge_queue
method to delete all messages from the Queue.
def purge_queue():
sqs_client = boto3.client("sqs", region_name="us-west-2")
response = sqs_client.purge_queue(
QueueUrl="https://us-west-2.queue.amazonaws.com/xxx/my-new-queue",
)
print(response)